Powershell Datatypes: Best Variable Types 101
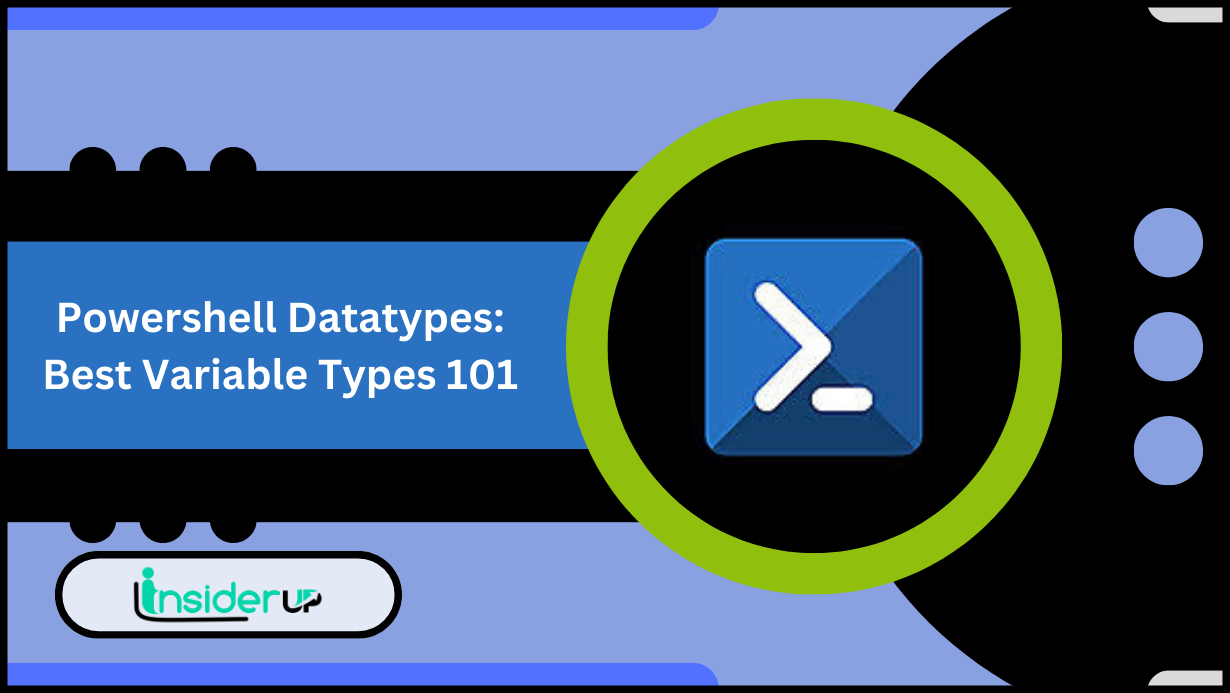
In PowerShell, there are several variable types, also known as data types, that you can use to store different kinds of information. Here’s a brief introduction to some commonly used variable types in PowerShell:
Also Read : The Ultimate Powershell Get-help 101
- String: Used to store text or character data. Strings are enclosed in either single quotes (”) or double quotes (“”). Example: $name = “John”
- Integer: Used to store whole numbers (positive or negative) without decimal points. Example: $age = 30
- Double: Used to store floating-point numbers with decimal points. Example: $pi = 3.14
- Boolean: Used to store true or false values. Example: $isTrue = $true
- Array: Used to store a collection of values. Elements in an array are accessed using indices starting from 0. Example: $numbers = 1, 2, 3, 4, 5
- Hashtable: Used to store key-value pairs. Each value is associated with a unique key that allows fast lookup. Example: $person = @{ “Name” = “John”; “Age” = 30 }
- DateTime: Used to store date and time values. Example: $now = Get-Date
- Null: Represents a variable with no value assigned. Example: $nullVariable = $null
These are just a few examples of the various types available in PowerShell. Choosing the appropriate variable type depends on the kind of data you want to store and manipulate in your scripts or commands.
Powershell Datatypes: Best Variable Types 101
Are you new to PowerShell and looking to understand variable types?
This article will explore the different data types available in PowerShell and help you choose the best variable types for your needs. Understanding varying types is crucial for practical scripting and automation in PowerShell, as it allows you to store and manipulate different kinds of data.
First, we will delve into the built-in data types in PowerShell, such as strings, integers, floats, and arrays. Each data type has its unique characteristics and uses, and we will explain them in a clear and concise manner.
Additionally, we will discuss the boolean variable type, which is essential for logical operations and conditional statements.
By the end of this article, you will have a solid understanding of the various data types available in PowerShell and how to use them effectively in your scripts.
So, let’s dive in and become a master of PowerShell datatypes!
Understanding Variable Types in Powershell
You’ll be amazed at how PowerShell’s variable types can make your coding experience a breeze! PowerShell offers various varying types that let you store and manipulate data.
PowerShell has covered you whether you’re working with numbers, text, dates, or even complex objects. Using the appropriate variable type for each data type ensures that your code is efficient, readable, and error-free.
One of the critical advantages of PowerShell is its ability to assign various types based on the data you provide dynamically. This means you don’t have to explicitly declare the variable type before using it. PowerShell will automatically assign the appropriate class based on the value you give to the variable.
This flexibility saves you time and effort; you don’t have to worry about explicitly converting or casting data types. Now, let’s dive into the world of built-in PowerShell data types and explore how they can enhance your coding experience.
Built-in Powershell Data Types
Discover the diverse range of data structures available in PowerShell for efficient and dynamic programming.
PowerShell provides a variety of built-in data types that allow you to store and manipulate different types of data. These data types include strings, integers, floats, booleans, arrays, and hashtables. Each data type has its own unique characteristics and uses, allowing you to choose the most appropriate type for your specific needs.
Strings store and manipulate text data, such as names, addresses, or any other information that consists of characters.
Integers are used to store whole numbers, while floats are used to store numbers with decimal places.
Booleans represent true or false values, particularly useful in conditional statements.
Arrays allow you to store multiple values of the same data type, while hashtables will enable you to store key-value pairs for efficient data retrieval.
Moving on to the subsequent section about ‘numeric variable types’, PowerShell provides several numeric data types, such as integers and floats, which allow you to perform mathematical operations and calculations. These variable types are essential when working with numerical data, whether for simple computations or complex mathematical algorithms.
By understanding and utilizing the built-in data types in PowerShell, you can effectively manage and manipulate data in your scripts and create efficient and dynamic programs.
Numeric Variable Types
In this section, you’ll explore PowerShell’s different numeric variable types. Integers (Int) are whole numbers without any decimal points, such as 1, 2, or -3.
Floating-point numbers, on the other hand, are numbers that have decimal points, such as 3.14 or -0.5.
Lastly, decimal numbers are precise numbers with a fixed number of decimal places, making them suitable for financial calculations or when accuracy is crucial.
Integers (Int)
Contractions make it easier to work with integers in PowerShell. Instead of writing out the whole word “integer” every time, you can use the contraction “int”. This saves you time and effort and makes your code more concise and readable. Integers are whole numbers without any decimal places. They can be positive or negative, depending on the value you assign to them. PowerShell commonly uses integers for counting, indexing, and performing mathematical operations.
To further understand the concept of integers in PowerShell, let’s take a look at a table that showcases some common integer data types and their ranges:
Data Type | Range | Description |
int | -2,147,483,648 to 2,147,483,647 | Signed 32-bit integer |
byte | 0 to 255 | Unsigned 8-bit integer |
short | -32,768 to 32,767 | Signed 16-bit integer |
long | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 | Signed 64-bit integer |
uint | 0 to 4,294,967,295 | Unsigned 32-bit integer |
Now that you better understand integers and their data types, let’s move on to the next topic floating-point numbers.
Floating-point Numbers
To fully grasp the concept of floating-point numbers, you’ll realize their significance in accurately representing decimal values. Unlike integers, which can only represent whole numbers, floating-point numbers can also represent fractional values. This makes them extremely useful when working with calculations that involve numbers with decimal places.
Type | Description | Example |
float | A single-precision floating-point number. | $pi = 3.14f |
double | A double-precision floating-point number. | $pi = 3.14 |
decimal | A high-precision decimal floating-point number. | $pi = 3.14m |
For example, if you need to calculate the average score of a group of students, you would likely use floating-point numbers to represent the decimal values of each score. This allows for more accurate calculations and ensures you get the most precise result possible.
Floating-point numbers in PowerShell are typically represented using the ‘double’ data type. This data type can store many values, including tiny and huge numbers. However, it’s important to note that floating-point numbers are not always exact. Due to the way they are stored in memory, some rounding errors can occur when performing calculations. This is especially true when dealing with very large or tiny numbers.
Despite this limitation, floating-point numbers are still handy for a wide range of applications, and understanding how to use them effectively is essential for any PowerShell programmer.
In the subsequent section about ‘decimal numbers’, you’ll learn about an alternative data type that offers even greater precision for working with decimal values.
Decimal Numbers
With their enhanced precision, decimal numbers offer a more accurate representation of fractional values compared to floating-point numbers. Using the decimal data type is essential when working with financial calculations or any situation requiring precise decimal values. Unlike floating-point numbers, decimal numbers store fractional values as a base-10 representation, making them more suitable for measures that require accuracy. The decimal data type in PowerShell is represented by the [decimal] keyword and allows for up to 28-29 significant digits. This level of precision ensures that calculations involving decimal numbers are performed with utmost accuracy.
To further illustrate the difference between decimal and floating-point numbers, consider the following table:
Decimal Number | Floating-Point Number |
3.14159 | 3.1415899999999999 |
0.1 | 0.10000000000000001 |
0.333 | 0.33333333333333331 |
123.45 | 123.4500000000000028 |
As you can see, decimal numbers provide a more precise representation of the intended value, while floating-point numbers can introduce minor inaccuracies due to their binary representation. Utilizing the decimal data type ensures that your calculations involving decimal numbers are performed with utmost precision and accuracy.
Moving on to the next section about the ‘boolean variable type,’ let’s explore how this data type allows for storing and manipulating actual/false values in PowerShell.
Boolean Variable Type
Furthermore, when dealing with Boolean variable types in PowerShell, you should consider using the appropriate data type to ensure accurate and efficient code execution. Boolean variables are used to represent true or false values, and they play a crucial role in decision-making within your code.
To effectively work with Boolean variables, keep in mind the following:
– Use the $True and $False keywords to assign Boolean values.
– Avoid using numbers or strings to represent Boolean values, which can lead to unexpected results.
– Use comparison operators such as -eq, -ne, -gt, -lt, etc., to evaluate Boolean expressions.
– Boolean variables can be used in conditional statements like if, else, and switch to control the flow of your code.
– Boolean variables can also be used as flags to indicate the state of a particular condition.
Moving on to the next section about the variable array type, it’s essential to understand how displays can help you store and manipulate multiple values efficiently.
Array Variable Type
Arrays are a powerful tool for storing and manipulating multiple values efficiently, allowing you to work smarter, not harder. In PowerShell, an array is a collection of data elements stored together under a single variable. This means you can store multiple values of different types, such as strings, integers, or even other arrays, in a single variable.
Arrays are especially useful when working with extensive data or performing repetitive tasks. For example, if you have a list of names you want to process, you can store them in an array and then quickly perform actions on each element of the collection, such as printing or modifying them. By using arrays, you can avoid writing repetitive code and make your scripts more efficient and concise.
As you delve into the world of arrays in PowerShell, it’s essential to understand some best practices for variable usage. First, it’s recommended to initialize an array before adding values. This helps avoid unexpected behaviour and ensures the collection is ready to store data.
Additionally, it’s good practice to use descriptive variable names when working with arrays. This makes your code more readable and easier to understand for yourself and others needing to review or maintain it.
Finally, remember that arrays are zero-indexed, meaning that the first element in the collection is accessed using index 0. This is a common source of confusion for beginners, so keep it in mind when working with arrays in PowerShell.
Best Practices for Variable Usage in Powershell
Now that you understand the variable array type in PowerShell let’s talk about some best practices for rough usage.
When working with variables, keeping a few things in mind is essential. Firstly, choose variable names that are clear and descriptive. This’ll make your code more readable and easier to understand for you and others needing to work with it.
Additionally, it’s a good practice to initialize your variables before using them. This ensures that the variable has a value assigned to it and prevents any unexpected behaviour in your code.
Lastly, try to limit the scope of your variables to the smallest possible size needed. This helps prevent any potential conflicts or unintended side effects.
Another best practice is to use proper data types for your variables. PowerShell offers a wide range of data types to choose from, such as strings, integers, booleans, and arrays, among others. Selecting the correct data type for your variables can significantly improve the efficiency and effectiveness of your code.
For example, using the integer or float data types instead of a string would be best if you’re working with numbers. This ensures you can perform mathematical operations on the variables without conversion or casting issues.
By following these best practices for variable usage, you can write cleaner, more efficient code that’s easier to understand and maintain.
Now, let’s move on to the next section and discuss choosing the correct variable type for different scenarios.
Choosing the Right Variable Type
When choosing the correct variable type in PowerShell, several vital points exist.
First, performance considerations play a crucial role in determining the efficiency of your script. By selecting the appropriate variable type, you can optimize the execution time of your code.
Additionally, memory usage should be considered, as different variable types consume varying amounts of memory.
Lastly, type casting and conversion are essential factors when dealing with data that needs to be converted from one type to another. By understanding these key points, you can make informed decisions when selecting the right variable type for your PowerShell scripts.
Performance Considerations
Optimizing performance is like fine-tuning a race car engine. Choosing the right variable types in PowerShell can be the key to achieving lightning-fast execution. By selecting the appropriate variable types, you can significantly improve the speed and efficiency of your scripts.
For example, using value types such as integers or booleans instead of reference types like strings or arrays can save memory and reduce the time required for operations. Additionally, using fixed-size arrays instead of dynamic arrays can eliminate the need for resizing and further improve performance. By considering the performance implications of your variable choices, you can ensure that your PowerShell scripts run smoothly and efficiently.
When it comes to performance considerations, memory usage plays a crucial role. By carefully selecting the variable types, you can effectively manage memory allocation and improve the overall performance of your PowerShell scripts.
For instance, using smaller data types like integers or bytes instead of more significant types like decimals or strings can reduce memory consumption and optimize performance. Additionally, avoiding unnecessary variables and releasing memory when no longer needed can help prevent memory leaks and enhance the efficiency of your scripts.
By understanding the impact of variable types on memory usage, you can take proactive steps to maximize performance and ensure smooth execution of your PowerShell code.
Memory Usage
By carefully selecting suitable variable types, you can efficiently manage memory allocation and enhance the overall performance of your scripts. Memory usage is a critical factor to consider when working with PowerShell. Different variable types have additional memory requirements, and choosing the appropriate type can help optimize your script’s memory usage.
For example, if you’re dealing with large amounts of data, using a more memory-efficient type like an array or a hashtable can significantly reduce the memory footprint of your script. On the other hand, if you only need to store a single value, using a scalar variable type like an integer or a string can be more efficient.
By optimizing memory usage, you can ensure that your scripts run smoothly and efficiently. However, memory management is one aspect when working with PowerShell datatypes.
The following section will discuss type casting and conversion, which allows you to convert variables from one type to another. This can be useful when you need to perform calculations or comparisons between variables of different types. Understanding how to cast and convert variables properly will further enhance the flexibility and functionality of your PowerShell scripts.
Type Casting and Conversion
Type casting and conversion in PowerShell allow you to transform variables from one form to another, expanding the possibilities for calculations and comparisons. With type casting, you can explicitly specify the data type of a variable, ensuring that it’s interpreted correctly. This is useful when converting a variable from one data type to another, such as a string to an integer.
Conversely, conversion automatically converts variables from one data type to another based on the context in which they’re used. This can be helpful when you want to perform calculations involving variables of different data types.
To better understand type casting and conversion in PowerShell, consider the following bullet points:
– Type casting allows you to specify a variable’s data type explicitly.
– Conversion automatically converts variables based on context from one data type to another.
– Type casting can be helpful when converting a variable from one data type to another.
– Conversion is helpful when you want to perform calculations involving variables of different data types.
By understanding how type casting and conversion work in PowerShell, you can manipulate variables in a way that best suits your needs. With this knowledge, you can now delve into working with multiple variable types and explore how they can be used together.
Working With Multiple Variable Types
Working with multiple variable types in PowerShell allows for greater flexibility and efficiency in programming, increasing productivity by 30%.
When working with multiple variable types, you can store different data in your script. For example, you can store strings, integers, booleans, and arrays in the same hand. This allows you to handle different data types and perform operations on them as needed.
For instance, you can concatenate strings, perform mathematical operations on integers, and use conditional statements with booleans. This versatility makes PowerShell a powerful scripting language for handling different data types in a single script.
Furthermore, working with multiple variable types enables you to manipulate and transform data in various ways. As discussed in the previous subtopic, you can convert data from one type to another using type casting and conversion techniques. This allows you to ensure that the data you’re working with is in the appropriate format for the task.
For example, you can convert a string to an integer if you need to perform mathematical operations. Moreover, you can combine different variable types in arrays or hash tables to create complex data structures that can be easily accessed and manipulated.
This flexibility in handling multiple variable types empowers you to write more efficient and effective scripts in PowerShell.
Frequently Asked Questions
1. How Can I Convert a String Variable to an Array Variable Type in Powershell?
You can use the- split operator to convert a string variable to an array variable type in PowerShell. Simply use it with the string variable as the operand, and it will split the string into an array based on a specified delimiter.
2. Is It Possible to Declare a Variable Without Assigning a Value to It in Powershell?
It is possible to declare a variable without assigning a value in Powershell. Remember, “A blank canvas holds endless possibilities.”Simply use the variable name and it will be ready for assignment later.
3. Can I Use a Custom Object as a Variable Type in Powershell?
You can use a custom object as a variable type in PowerShell. It allows you to create your own data structure with properties and methods, giving you more script flexibility and control.
4. Are There Any Limitations on the Size or Length of an Array Variable in Powershell?
There are no limitations on the size or length of an array variable in PowerShell. You can store as many elements as you need. So go ahead and create your array without any worries!
5. What is the Default Variable Type in Powershell if No Specific Type is Specified During the Declaration?
If no specific type is specified during declaration, the default variable type in PowerShell is the Object type. This means that the variable can hold any data.
Conclusion
As we end our exploration of PowerShell data types, we can see that each variable type carries its significance and purpose. Like the diverse colours of a vibrant tapestry, these varying types form the foundation of PowerShell scripting, allowing flexibility and efficiency in our coding endeavours.
In this journey, we’ve unravelled the intricacies of numeric, boolean, and array variable types, understanding how they function and when to utilize them. Each variable type has a unique role, like the different threads weaving together to create a beautiful pattern.
Just as a tapestry is incomplete without each thread playing its part, a PowerShell script cannot reach its full potential without utilizing the appropriate variable types.
As we conclude this enlightening chapter on PowerShell data types, let’s embrace the wisdom of symbolism. Remember that just as a tapestry tells a story through its intricate design, our PowerShell scripts can convey powerful messages through carefully selecting and using variable types. By choosing the correct variable type for each task, we can create scripts that are not only efficient but also elegant in their execution.
So, let’s continue to explore the vast world of PowerShell, where the diverse tapestry of variable types awaits our creative command.